Garmin Connect IQ build, assembly for automating simulator behavior.
- Functional overview
- How to use in each environment
- Other
- Change log
- Help
- class Checker
- interface IEnvironment
- class GarminSDK
- class Jungle
- class Simulator
- class TimeSimulator
- delegate Simulation
- delegate PrePostAction
- enum GoalType
- enum Activity
- enum BackgroundColor
- enum Language
- enum ConnectionType
- enum WiFiStatus
- enum ReceiveNotificationType
- enum GPSQualityType
- enum SettingToggleMenu
- class Usage<T>
- class MemoryDiagnostics
- enum ExecuteType
Functional overview
The following classes are prepared so that the program can be built, the simulator can be executed, and the simulator can be controlled from the program.
- Extract environment information for Connect IQ
GarminSDK Class
When an object is created, the SDK specified in Current by sdkmanager is read and the environment is set.
With the above settings, 3.2.3. - Loading and managing the project (monkey.jungle)
Jungle Class
A class for reading the Connect IQ Project on the Eclipse side and acquiring various information. Required for the Garmin SDK build process. - Simulator control
Simulator Class - Ability to manage classes 1 to 3 for ease of use
Checker Class
See help for an overview of the class and a description (details) of the method parameters.
How to use in each environment
Confirmed environment
- Visual Studio 2019 .Net 5.0
- PowerShell 7.1
When using with C # (Visual Studio 2019)
Search for “AutomationConnectIQ.Utility” in NuGet and install it.
After that, the class can be used in the namespace “AutomationConnectIQ.Utility”.
When using with PowerShell
Package installation
Install-Package automationconnectiq.utility
Load the package above.
Regarding the installation destination, it may be better to set it separately with the “-Scope” parameter.
For the current user only, I did the following:
Install-Package automationconnectiq.utility -Scope CurrentUser
Preparation for package use
With PowerShell, you can’t just install the package, you need to load the dll.
Basically, execute the following script in advance.
This script finds the location of the automationconnectiq.utility package and loads the dll under it.
Get-ChildItem -Filter *.dll -Recurse (Split-Path (Get-Package AutomationConnectIQ.Utility).Source) |
ForEach-Object { Add-Type -LiteralPath $_ }
Please write this in one of the following places.
- Describe it at the beginning of the file ps1.
Now, when you run ps1, you can load the dll and call the classes in AutomationConnectIQ.Utility from PowerShell. - Describe it in “% USERPROFILE% DocumentsPowerShellMicrosoft.PowerShell_profile.ps1”.
This file is loaded by default in the user environment when PowerShell 7.x starts, and by writing it here, the classes in AutomationConnectIQ.Utility can be called in all the environment of the user.
Other
Click here for sample programs.
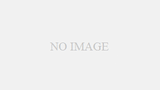
Click here for the source code.
Change log
Ver0.4.1
Additional features
- BuildOption settings.
Added a mechanism to pass optimization options added in Connect IQ 4.1.4 and 4.2.
Bug fixes
- Fixed an issue where the simulator title name was changed in Connect IQ 4.1.5 and the simulator could not be started correctly.
Since Connect IQ 4.1 changed the battery status setting method, it corresponds to it.
Corresponds to the change in the specification of the notification window in Connect IQ 4.2.
Ver0.3.3
Bug fixes
- The OK / Cancel command did not work on the “Edit Activity Monitor Info Screen”.
With Connect IQ 4.0.x, the UI seems to have changed and I couldn’t handle it.
Ver0.3.2
Additional features
- Added the function to set information in Active Monitor
- Added a function to execute Unit Test to Checker / Garmin SDK
- Pre-action can be specified in Checker.Check specified by device
- Added project device information acquisition function to Checker
- Addition of property whether HeatMap can be displayed
Bug fixes
- In some cases, GetMemoryDiagnostics could not get the value.
- Fixed a small code error in getting ON / OFF of the Toggle menu, which could cause malfunctions in functions that use this function in the future.
Ver0.2.1
Fixed the following bugs
- Abnormal termination when calling Simulator.GetMemoryDiagnostics.
- The value was not reflected in Simulator.SetNotificationCount.
Ver0.2.0
Initial version
The major version number is left at 0 because there are some operations that cannot be done for the Simulator class.
Also, continuous testing is done by Garmin SDK, Jungle, Time Simulator, but since only the one related to watch face is done about Simulator, it may not work at the time of version and revision upgrade.
0.1.x is a missing number.
Help
class Checker
Utility class that builds and checks automatically
Properties
-
String Key { set; }
Key file used in build
-
String Project { set; }
Project file used for build
(monkey.jungle) -
String LogFile { set; get; }
Log file for output
If Writer is non-null, that will take precedence -
StreamWriter Writer { set; }
Stream to output log file
-
List<String> Devices { get; }
Target device information
-
String BuildOption { get; }
BuildOption settings
Added in Ver0.4.1.
Methods
-
Boolean Check (System.String device,AutomationConnectIQ.Lib.Checker.Simulation func,AutomationConnectIQ.Lib.Checker.PrePostAction pre);
Perform build & check for the specified device
- device
Device name to be checked - sim
For simulator processing - pre
Device simulation pre-processing - returns:
Return true when simulation is complete
- device
-
Boolean Check (System.Boolean isBreak,AutomationConnectIQ.Lib.Checker.Simulation func,AutomationConnectIQ.Lib.Checker.PrePostAction pre,AutomationConnectIQ.Lib.Checker.PrePostAction post);
Check all devices
- isBreak
If you get an error on any one device, stop there - func
For simulator processing - pre
Device simulation pre-processing - post
Device simulation post-processing
- isBreak
-
Boolean UnitTest (System.String device);
Implementation of Unit Test
- device
Device name to process
- device
interface IEnvironment
Extract environment information for Connect IQ
Properties
-
String AppBase { get; }
Connect IQ storage information
class GarminSDK
Classes for handling Connect IQ related files
Constructors
-
GarminSDK ();
Default constructor
-
GarminSDK (AutomationConnectIQ.Lib.IEnvironment env);
constructor
Properties
-
String SdkFolder { get; }
Folder name of the current SDK
-
String Version { get; }
Current SDK version
-
String Key { set; }
Key file used in build
- remarks:
If not set, it will always be false in the build
- remarks:
-
StreamWriter Writer { set; }
Output redirect destination for simulator startup, build, etc.
- remarks:
Be sure to set this to null before closing the set Writer.
- remarks:
Methods
-
Process StartSimUI ();
Running the simulator
- remarks:
If there is something in the Writer, redirect the output to it.
Use Simulator.WaitForInput to wait for the completion of startup to enable operations such as simulator menus.
- remarks:
-
Void StartProgram (System.String progName,System.String device,System.Boolean isUnitTest);
Run the program in simulation
- progName
program name - device
Device name - isUnitTest
Set to true when executing Evil UnitTest program
Operates with false if no argument is specified - remarks:
Use WaitForDeviceStart to wait for the watch program to finish starting in the simulator.
- progName
class Jungle
Analyze Connect IQ project files to enable various processing
remarks:
-Allow the program name and device name used to be retrieved.
-Continuously execute the simulation
Constructors
-
Jungle (System.String projectFile);
Project loading
Exceptions may return the same as StreamReader- projectFile
Project file name
- projectFile
Properties
-
List<String> Devices { get; }
Target device information
-
String EntryName { get; }
Entry name described in the project
(The entry of iq: application is output)- remarks:
The real build-time name is in the name of iq: application, which refers to the resource.
I haven’t looked at the resources in this class, so I haven’t referenced their names.
- remarks:
-
String JungleFile { get; }
Project file name
-
String DefaultProgramPath { get; }
Default program output file name
- remarks:
ProgramName under bin in the location of the jungle file with the extension “prg”
- remarks:
Methods
-
static Jungle Create (System.String projectFile);
Factory function to create Jungle from project file
If the analysis method is different due to different versions, try to absorb them here- projectFile
Target project name File name (specify monkey.jungle)
- projectFile
-
Boolean IsValidDevice (System.String device);
Determine if the specified device name is registered
-
static String MakeProgramPath (System.String name);
Make a program name
Actually I just added the extension
class Simulator
Simulator operation
Constructors
-
Simulator ();
Default constructor
-
Simulator (AutomationConnectIQ.Lib.GarminSDK sdk);
constructor
Properties
-
Boolean IsEnabledHeatMap { get; }
Returns whether the View Screen Heat Map is selectable
If true, note that Heat Map will be displayed when Low Power Mode is set to True.
Methods
-
Void Open (AutomationConnectIQ.Lib.GarminSDK sdk);
Launching the simulator
If it’s already running, look for it, but if it’s not, start it yourself -
Void WaitForInput ();
After calling GarminSDK.StartSimUI, wait until the program load on the simulator side is completed
Just wait until Ready appears in the status bar. -
Void Close ();
Exit the running simulator
-
Void KillDevice ();
End the simulation of the booting device
The simulator itself does not close
A function to be executed before loading a different program device. -
TimeSimulator CreateTime ();
Generation of time simulation class
-
ActivityMonitor CreateActivityMonitor ();
Generate Activity Monitor class
-
Void TriggerGoal (AutomationConnectIQ.Lib.Simulator.GoalType type);
Goal notification
-
Void SetTimerActivity (AutomationConnectIQ.Lib.Simulator.Activity type);
Click the Data Fields-Timer menu.
-
Boolean IsEnableTimerActivity (AutomationConnectIQ.Lib.Simulator.Activity type);
Data Fields-Timer menu state.
-
Void SetBackgroundColor (AutomationConnectIQ.Lib.Simulator.BackgroundColor type);
Data Fields background color settings.
-
Boolean SetLanguage (AutomationConnectIQ.Lib.Simulator.Language lang);
UI language settings
-
Void SetBleConnection (AutomationConnectIQ.Lib.Simulator.ConnectionType type);
BLE connection type setting
-
Void SetWiFiConnection (AutomationConnectIQ.Lib.Simulator.ConnectionType type);
WIFI connection type setting
-
Void SetWiFiStatus (AutomationConnectIQ.Lib.Simulator.WiFiStatus type);
WIFI status settings.
-
Void SetDisplayHourType (System.Boolean is24Type);
Time notation setting.
- is24Type
If true, it is written in 24 hours, if it is false, it is written in 12 hours.
- is24Type
-
Void SetDisplayUnit (System.Boolean isMetric);
Display unit setting.
- isMetric
If true, it is expressed in meters, and if false, it is expressed in miles.
- isMetric
-
Void SetFirstDayWeek (System.DayOfWeek type);
Setting the day of the week to be the beginning of the week.
- type
Specify the day of the week only on Saturday, Sunday, and Monday
- type
-
Void SetReceiveNotificationType (AutomationConnectIQ.Lib.Simulator.ReceiveNotificationType type);
Set the notification type to receive
-
Void SetGPSQuality (AutomationConnectIQ.Lib.Simulator.GPSQualityType type);
Set GPS quality type
-
Void SetNotificationCount (System.Int32 num);
Set the number of notifications
- num
Number of notifications(0-20)
- num
-
Void SetAlarmCount (System.Int32 num);
Set the number of alarms
- num
alarms(0-3)
- num
-
Void SetBatteryStatus (System.Double chargingRate,System.Boolean isCharging);
Set the charge status and charge rate of the battery
-
Boolean SetBleSettings (System.String portName);
Set the BLE connection port
- returns:
False if the port cannot be connected.
However, if you enter the same port name consecutively, it will be true the second time, so be careful.
- returns:
-
Void SetGPSPosition (System.Double latitude,System.Double longitude);
Set GPS coordinates.
For the coordinate values, you can set the two real values that appear at the bottom of the screen as they are when you click on google map. -
Boolean SetForceOn (System.Boolean toHide);
Settings-> Force on ???? settings
- toHide
True if onHide is selected, false if onShow is selected - returns:
False if not selected
- toHide
-
Void ToggleMenu (AutomationConnectIQ.Lib.Simulator.SettingToggleMenu type,System.Boolean turnOn);
Set menu toggle ON / OFF
-
Void FastForward (System.UInt32 miniuete);
Advance time with Activity Monitor settings
- miniuete
Time to advance (minutes: 1-600)
- miniuete
-
TimeDiagnostics GetTimeDiagnostics (System.Boolean doClose);
Get the contents of the time measurement screen (Watchface Diagnostics)
- doClose
Set to true to close open Watchface Diagnostics - returns:
Watchface Diagnostics settings
- doClose
-
MemoryDiagnostics GetMemoryDiagnostics ();
Retrieving memory information
Sometimes it fails.- returns:
If it is null, it has failed, so you may be able to get it by retrying.
- returns:
-
Void WaitForDeviceStart ();
Wait for the device to boot up
class TimeSimulator
Time simulation window control class
Properties
-
DateTime Time { set; }
Time setting
-
UInt32 Factor { set; get; }
Setting the progress rate
-
Boolean IsOpen { get; }
Whether the time simulation window is open
-
Boolean IsStarted { get; }
Whether the time simulation is running
Methods
-
Void Open ();
Open the time setting window
-
Void Close ();
Close the time setting window
- remarks:
When the time simulation is started, it is stopped and then closed.
- remarks:
-
Void Action (AutomationConnectIQ.Lib.TimeSimulator.ExecuteType type);
Press the button to perform a time simulation.
- remarks:
The screen may not be updated until Sleep (500ms) is reached after Start.
Be careful when implementing.
- remarks:
delegate Simulation
Delegate processing of the simulation part used in the check
delegate Boolean Simulation(System.String device,AutomationConnectIQ.Lib.Simulator sim);
delegate PrePostAction
Delegate processing of the processing part performed before and after the check
Mainly for making the simulator preset etc.
delegate Void PrePostAction(AutomationConnectIQ.Lib.Simulator sim);
enum GoalType
Types of goal notifications
Fields
- Steps
- FloorClimbed
- ActiveMinutes
enum Activity
Data Fields-Timer settings
Fields
- Discard
- Save
- Pause
- Resume
- Start
- Lap
- WorkoutStep
- NextMultisport
enum BackgroundColor
Data Fields background color
Fields
- White
- Black
enum Language
Language setting
Fields
- Arabic
- Bulgarian
- Czech
- Danish
- German
- Dutch
- English
- Estonian
- Finnish
- French
- Greek
- Hebrew
- Croatian
- Hungarian
- Italian
- Latvian
- Lithuanian
- Norwegian
- Polish
- Portuguese
- Romanian
- Russian
- Slovak
- Slovenian
- Spanish
- Swedish
- Turkish
- Ukrainian
- BahasaIndonesia
- BahasaMalaysia
- ChineseSimplified
- ChineseTraditional
- Japanese
- Korean
- Thai
- Vietnamese
enum ConnectionType
BLE / WIFI connection type
Fields
- NotInitialized
- NotConnected
- Connected
enum WiFiStatus
WIFI status
Fields
- Avaliable
- LowBattery
- NoAccessPoints
- Unsupported
- UserDisabled
- BatterySaverActive
- StealthModeActive
- AirplaneModeActive
- PoweredDown
- Unknown
enum ReceiveNotificationType
Notification type to accept
Fields
- All
- Actionable
- ActionableLTE
- None
enum GPSQualityType
GPS quality type
Fields
- NotAvailable
- LastKnown
- Poor
- Usable
- Good
enum SettingToggleMenu
Toggle menu to turn on / off
Fields
- Tones
- Vibrate
- SleepMode
- ActivityTracking
- DoNotDisturb
- UseDeviceHTTPSRequirements
- LowPowerMode
- AppLockEnabled
- EnableAlert
class Usage<T>
A structure that holds the current, maximum, and peak values for use in Memory Diagnostics
Typeparameters
- T
Value type. It assumes int or double.
Fields
-
T Current
Current
-
T Max
Max
-
T Peak
Peak
class MemoryDiagnostics
Memory usage information
Fields
-
Usage<Double> Memory
Information about memory
-
Usage<Int32> Objects
Information about the object
enum ExecuteType
Types of buttons to press
Fields
- Start
- Stop
- Pause
- Resume